同様の記事もままありますが、この記事はお決まりの構成として使える状態までの手順です。
React + Vite にした理由は、webpack をこれから新規に0ベースで採用していくのは微妙、React なら Next.js が早く使えそうだけど、フレームワークはやはり依存度が大きい(Gatsby しかり、2-3年で移り変わりが激しく長く使うプロダクトには向かない)。Vite は webpack → vite への移行記事も多くあり、将来別のものになっても移行しやすい(はず)。
バージョン
% npm list --depth=0
├── @types/react-dom@18.0.9
├── @types/react@18.0.26
├── @typescript-eslint/eslint-plugin@5.46.0
├── @typescript-eslint/parser@5.46.0
├── @vitejs/plugin-react@3.0.0
├── eslint-config-prettier@8.5.0
├── eslint-plugin-react-hooks@4.6.0
├── eslint-plugin-react@7.31.11
├── eslint@8.29.0
├── prettier-plugin-organize-imports@3.2.1
├── prettier@2.8.1
├── react-dom@18.2.0
├── react@18.2.0
├── typescript@4.9.4
└── vite@4.0.0
プロジェクトの作成
// react typescript のプロジェクト作成
yarn create vite react-vite --template react-ts
// 依存関係インストール
yarn
// 起動
yarn dev
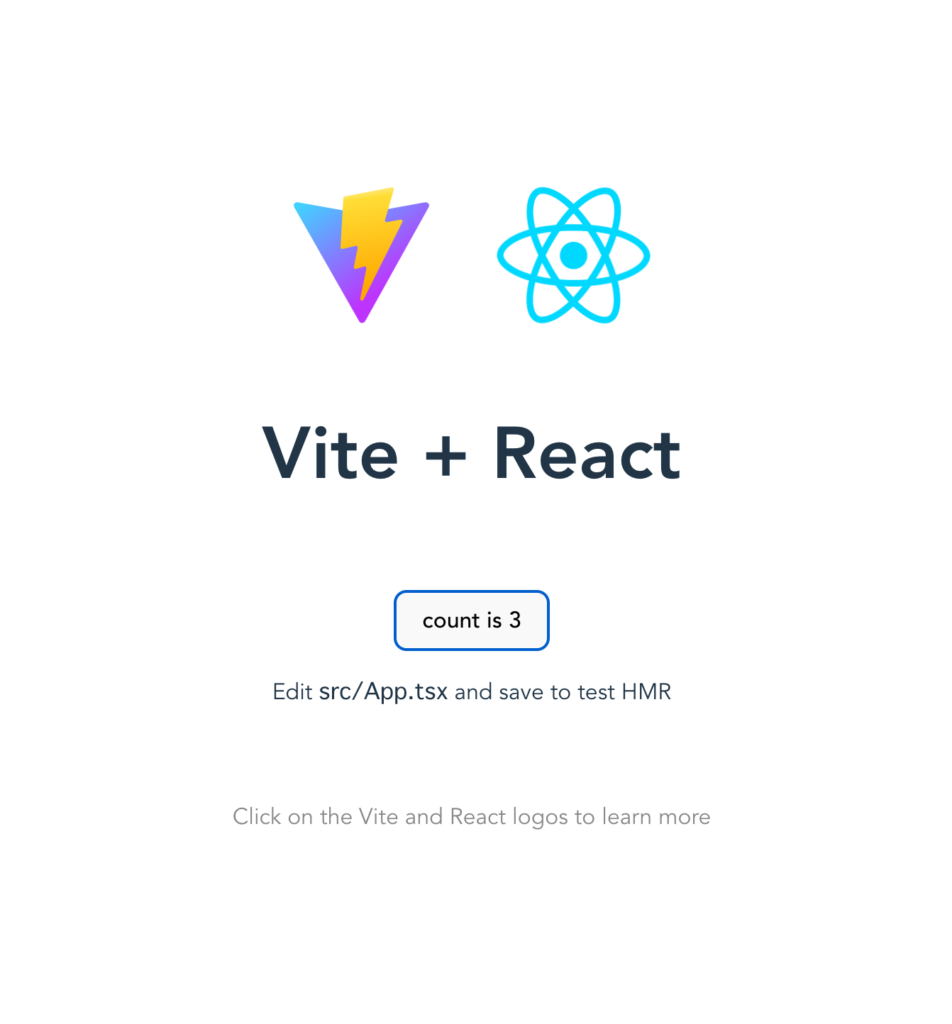
Eslint & Prettier
恒例のやつですね。
ただ、eslint-plugin-prettier は 2022/12時点では推奨されていないようです。prettier-eslint もです。なので、以下が主流とのこと。
- eslint と prettier はそれぞれ使い、eslint は静的解析、prettier はフォーマットとして使う。
- eslint のフォーマット機能は eslint-config-prettier でオフにする。
それぞれ設定していきます。
Eslint
以下で対話的にできるようです。
% yarn create @eslint/config
フロント開発だとだいたいみんな同じになるんじゃないかと思います。
ファイルフォーマットだけが好みですかね。スッキリ & コメントが書ける yaml にします。
% yarn create @eslint/config
success Installed "@eslint/create-config@0.4.1" with binaries:
- create-config
✔ How would you like to use ESLint? · problems
✔ What type of modules does your project use? · esm
✔ Which framework does your project use? · react
✔ Does your project use TypeScript? · No / Yes
✔ Where does your code run? · browser
✔ What format do you want your config file to be in? · YAML
Local ESLint installation not found.
The config that you've selected requires the following dependencies:
eslint-plugin-react@latest @typescript-eslint/eslint-plugin@latest @typescript-eslint/parser@latest eslint@latest
✔ Would you like to install them now? · No / Yes
✔ Which package manager do you want to use? · yarn
Installing eslint-plugin-react@latest, @typescript-eslint/eslint-plugin@latest, @typescript-eslint/parser@latest, eslint@latest
結果、生成されるものは以下のファイルです。
env:
browser: true
es2021: true
extends:
- eslint:recommended
- plugin:react/recommended
- plugin:@typescript-eslint/recommended
overrides: []
parser: '@typescript-eslint/parser'
parserOptions:
ecmaVersion: latest
sourceType: module
plugins:
- react
- '@typescript-eslint'
rules: {}
package.json に追加されたのは以下です。
"devDependencies": {
...
"@typescript-eslint/eslint-plugin": "^5.46.0",
"@typescript-eslint/parser": "^5.46.0",
"eslint": "^8.29.0",
"eslint-plugin-react": "^7.31.11",
...
}
Prettier
インストールするだけです。
yarn add --dev prettier eslint-config-prettier
設定ファイルは手動で用意しました。
tabWidth: 2
singleQuote: true
trailingComma: all
semi: false
useTabs: false
必須 追加設定
その1:eslint-config-prettier を .eslintrc.yml に指定
extends:
- ...
- prettier #eslint-config-prettier
その2:react/react-in-jsx-scope: off を .eslintrc.yml に設定
このまま lint すると、`’React’ must be in scope when using JSX のエラーがでますが、現在は
import React from ‘react’ は不要になったとのことなので、rule に追加します。
rules:
react/react-in-jsx-scope: off
その3:react のバージョンを .eslintrc.yml に設定
lint を実行すると以下の警告もでます。github に書いてあるように設定の追加をします。
Warning: React version not specified in eslint-plugin-react settings. See https://github.com/jsx-eslint/eslint-plugin-react#configuration .
settings:
react:
version: detect
その4:package.json にスクリプトを追加
最後に、lint で eslint と prettier 両方を実行するようにしています。
"scripts": {
...
"lint": "eslint --ext .js,.tsx,.ts ./src && prettier --write \"**/*.+(js|json|yml|ts|tsx)\""
}
実行
% yarn lint
yarn run v1.22.19
$ eslint --ext .js,.tsx,.ts ./src && prettier --write "**/*.+(js|json|yml|ts|tsx)"
.eslintrc.yml 40ms
.prettierrc.yml 4ms
package.json 42ms
src/App.tsx 186ms
src/main.tsx 7ms
src/vite-env.d.ts 4ms
tsconfig.json 5ms
tsconfig.node.json 4ms
vite.config.ts 6ms
✨ Done in 3.72s.
その他 追加設定
import の並び順 / 未使用 import の自動整列,削除
eslint-plugin-import や eslint-plugin-unused-imports もありますが、フォーマットは prettier の役割なので prettier-plugin-organize-imports
を使用します。これはインストールするだけでOKです。
yarn add --dev prettier-plugin-organize-imports
react hooks のルールチェック
yarn add --dev eslint-plugin-react-hooks
ymlファイルに追加します。
extends:
- eslint:recommended
- plugin:react/recommended
- plugin:react-hooks/recommended
- plugin:@typescript-eslint/recommended
- prettier #eslint-config-prettier
パスのエイリアス解決
import などのときに、@ などエイリアスが指定できるようにします。以下を追加することで、vite 側で alias を書かなくても、tsconfig の設定を見て解決してくれるようになります。
yarn add --dev vite-tsconfig-paths
plugin に追加します。
import react from '@vitejs/plugin-react'
import { defineConfig } from 'vite'
import tsconfigPaths from 'vite-tsconfig-paths'
// https://vitejs.dev/config/
export default defineConfig({
plugins: [react(), tsconfigPaths()],
})
あとは、tsconfig.json にエイリアス設定をします。
"compileOptions": {
...
"baseUrl": ".",
"paths": {
"@/*": ["src/*"]
},
}
Storybook
Storybook は公式にある通り以下でOKでした。
npx storybook init --builder @storybook/builder-vite
コメント